- Overview
In this article , we will learn to handle Dynamic Web Table in Selenium WebDriver .
2. Basic Concepts:
- A table with rows and columns on a web page is called a web table. In HTML, a table is created using tag.
- Table contains headers represented as, rows asand Coloums as tag
- There are two types of web Tables
- Static web table: Number of rows and columns will be static means constant that will not be changed. Here we just need to find the WebElement of the particular row or column and simply can perform operations on the cell.
- Dynamic table: Number of rows and columns will be dynamic. It will be kept on changing based on data and this the area where we will be focussing on.
3. Ways to Handle Dynamic WebTable:
- The standard way where we will iterate over the rows and columns and reach to a particular cell and get the cell value.
- By using custom XPath
Before proceeding further, let’s see how dynamic table looks like :
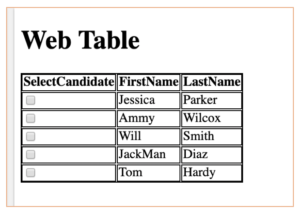
And below is the HTML representation of the above web table:
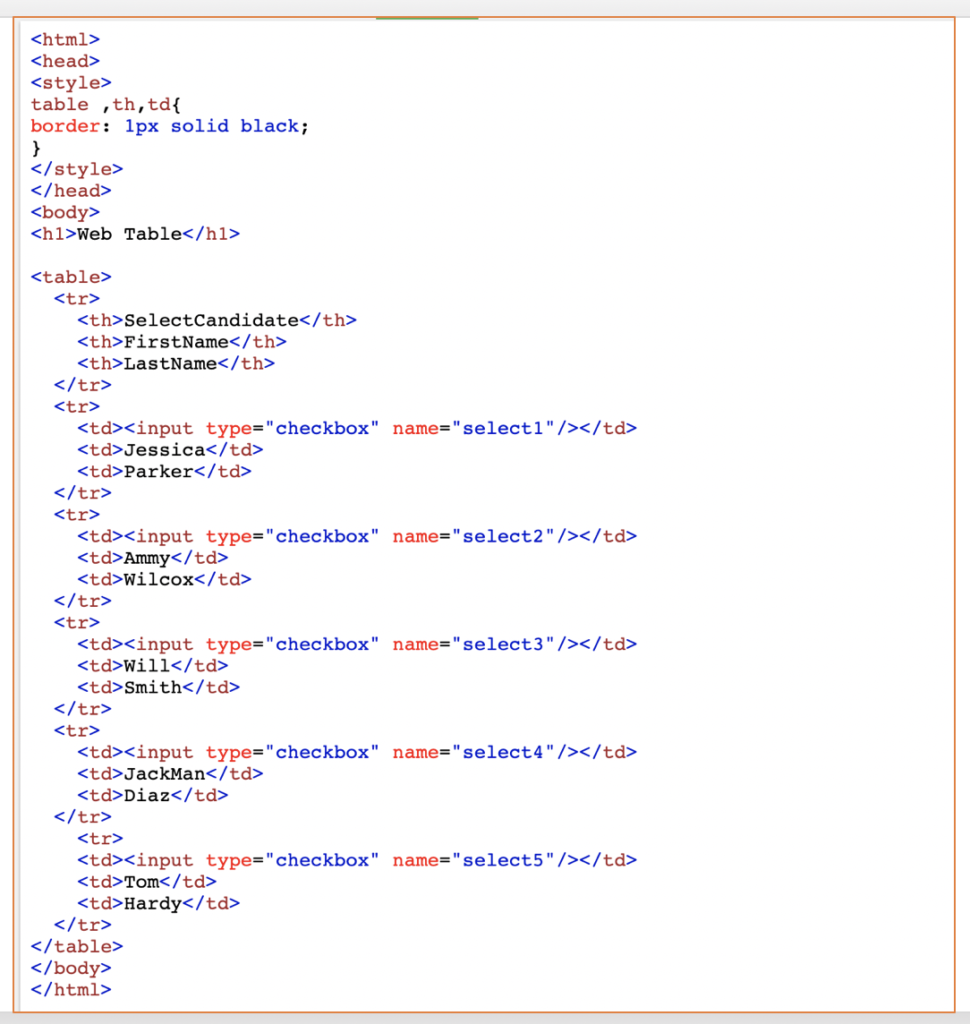
So, based on the data input we can increase or decrease our number of rows or columns.
Since we have seen what a web table is and how it is created, now let’s take the simple scenario :
1)click on checkbox where firstName is Will and remember it’s a dynamic table, hence “WILL” now on 3rd row can be shifted to other row based on the insertion or deletion of data.
- Let’s implement it by First Method:
Let’s accomplish this task first by looking at the code snippet below
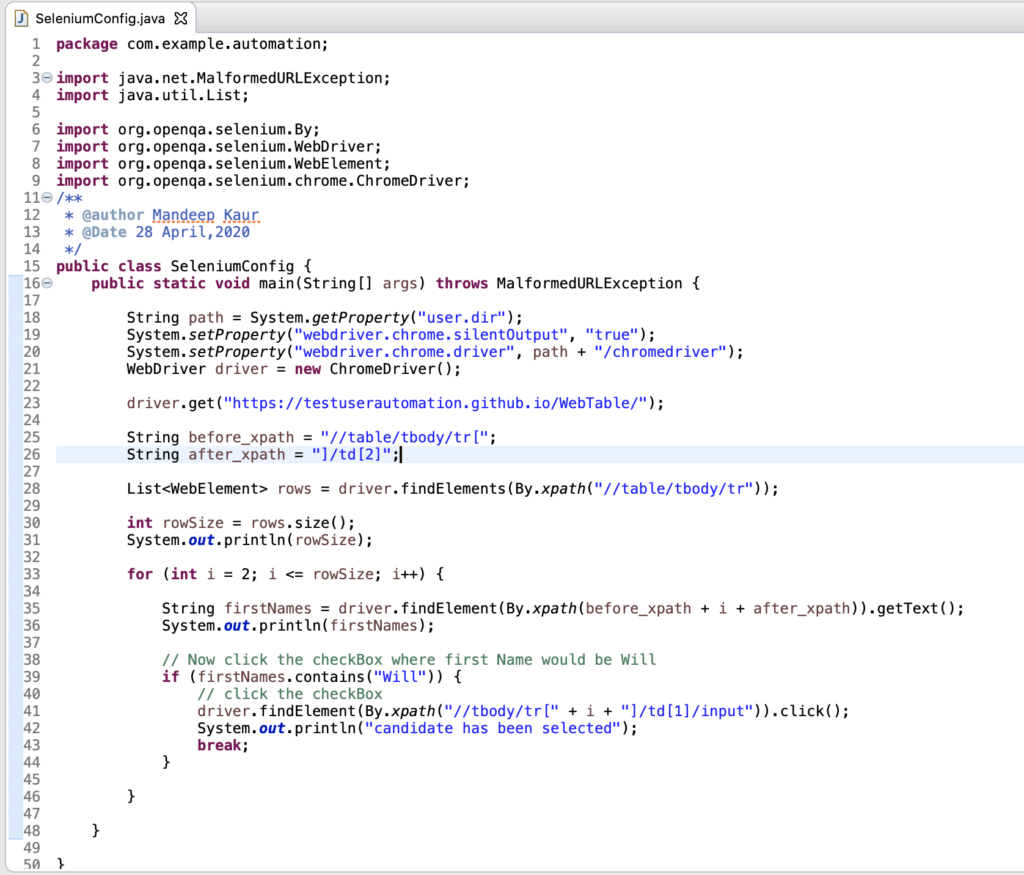
We’ll break this code into steps to get a deeper understanding of it:
Step1 : Capture xpath of the FirstName Row and observe the pattern
- First Row xpath: //table/tbody/tr[2]/td[2] –>jessica
- second Row xpath: //table/tbody/tr[3]/td[2] –>Ammy
- Third Row xpath: //table/tbody/tr[4]/td[2] –>Will and so on
- Here can we say that our rowis getting changed and our columnis constant.
Step2: Catch before and after XPath from the point our row started changing as done in the code.
Step 3: Now, we have captured the before and after XPath.Find out the total row size by size() function of the java collections.
Step 4: Start iterating over the rows, start the row index with 2 as the first row would be reserved for headers.
Step 5:Get the text of all the rows and store it to the variable firstNames and print it to see if we are able to capture it
Step 6 : Final step of our task , that click the checkbox where FirstName is Will
For this step, we put one condition that if firstNames contains WILL then only checkbox has to be clicked. if it exists it will click the checkbox prior to WILL.
Since WILL is existing in the table , it will click the checkbox and we can capture it from the browser:
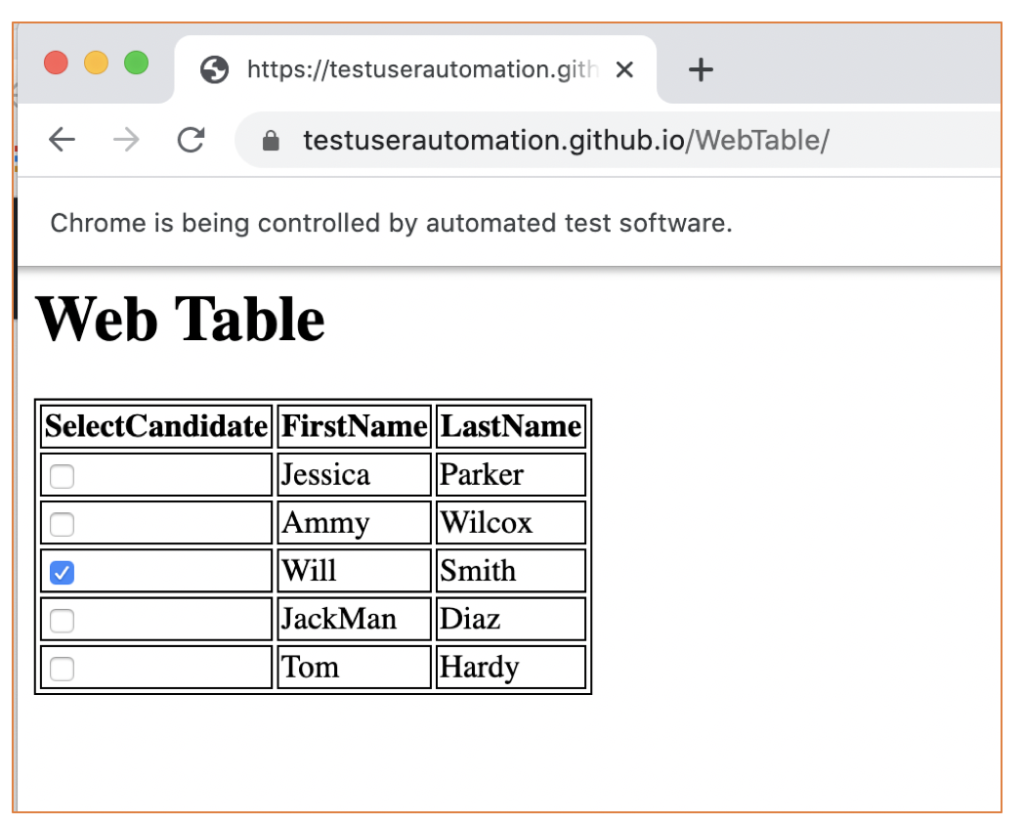
and our output on the console is similiar to following :
- By Second Method : with Custom XPath
We can achieve our task with single statement :
driver.findElement(By.xpath(“//td[contains(text(),’Will’)]//preceding-sibling::td//input[@type=’checkbox’]”)).click();
yes , that ‘it , below code for your reference
Let’s try to understand this method :
firstly visit over XPath_Axes , here you will get to know how to traverse from one node to another node.
Next, in the above we have spied over FirstName with text Will and we found out Will Sibling is td when we proceed upwards and then we get our input as a checkbox and from here we can simply capture it.
4. Which Method to choose
As can be conveyed from the above methods, the custom method is the best way to handle web dynamic table :
Reasons :
- In the First Method, it’s a lengthy method whereas the second method is just a single line statement
- In the first Method, we had to use for loop with conditions and then selecting the value, on the other hand, we need not have to use for loop
- As we are iterating through till all the rows it makes code slower in the first Method considering the other method can be seen fast, dynamic and efficient
Code Snippet with both methods :
package com.example.automation;
import java.util.List;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
/**
@author Mandeep Kaur
@Date 28 April,2020
*/
public class SeleniumConfig {
public static void main(String[] args) {
String path = System.getProperty("user.dir"); System.setProperty("webdriver.chrome.silentOutput", "true"); System.setProperty("webdriver.chrome.driver", path + "/chromedriver");
WebDriver driver = new ChromeDriver(); driver.get("https://testuserautomation.github.io/WebTable/");
// Method 1
String before_xpath = "//table/tbody/tr["; String after_xpath = "]/td[2]";
List rows = driver.findElements(By.xpath("//table/tbody/tr"));
int rowSize = rows.size();
System.out.println(rowSize);
for (int i = 2; i <= rowSize; i++) {
String firstNames = driver.findElement(By.xpath(before_xpath + i + after_xpath)).getText();
System.out.println(firstNames);
// Now click the checkBox where first Name would be Will
if (firstNames.contains("Will")) {
// click the checkBox
driver.findElement(By.xpath("//tbody/tr[" + i + "]/td[1]/input")).click(); System.out.println("candidate has been selected");
break;
}
}
// Method 2 : By custom xpath:
driver.findElement(By.xpath("//td[contains(text(),'Will')]//preceding-sibling::td//input[@type='checkbox']")) .click();
driver.close();
}
}
5. Conclusion
In this article , we learnt about how to handle dynamic Web Tables.
Cheers!!
Naveen AutomationLabs
Blog Contributors:
Author: Mandeep Kaur
Mandeep, having 5+ years of Testing experience in automation using Selenium (Java). Expertise in API and Performance testing using JMeter.
https://www.linkedin.com/in/mandeep-kaur-486ba9106
Reviewer: Naveen Khunteta
https://www.linkedin.com/in/naveenkhunteta
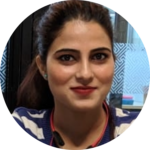