Steps to Push Code to Git Repositories using Command Prompt
Pre-requisites:
1. Download Git from below Link:
https://git-scm.com/download/win/ [Git must be installed in Local System].
2. Once Git is installed >> SSH Key should be Added in Git.
Login to Github >> Go to Settings >> User should be able to see SSH and GPG Keys on Left Side Panel as per below Screenshot.
Click on SSH and GPG Keys >> User should be able to see as shown below in same page.
Now Click on New SSH Key and Add SSH Key.
Below are the Steps to Generate SSH Key using Git bash:
Step1: Launch git bash.
Step2: Type ssh-keygen -t rsa -> Enter -> Enter -> Enter -> Key will be generated.
Admin@Admin-PC MINGW64 ~
$ ssh-keygen -t rsa
Generating public/private rsa key pair.
Enter file in which to save the key (/c/Users/Admin/.ssh/id_rsa):
/c/Users/Admin/.ssh/id_rsa already exists.
Overwrite (y/n)? y
Enter passphrase (empty for no passphrase):
Enter same passphrase again:
Your identification has been saved in /c/Users/Admin/.ssh/id_rsa.
Your public key has been saved in /c/Users/Admin/.ssh/id_rsa.pub.
The key fingerprint is:
SHA256:RWDx9MsZekrqrGMqKoL7tWVvjq51BSlUnzknk78RAzU Admin@Admin-PC
Now Go to Path as shown above which is highlighted.
[Path will be changed from System to System].
Open the File which is of Publisher type and user should be able to see SSH Key which needs to be added in Git.
[Make sure key is starting with ssh-rsa].
Once SSH key is added, you are good to add your code to created repository in Git.
Note: We can add as many as ssh keys, if we need to give permission for someone who can contribute to our repositories.
Below are the commands to Push Code from Command Prompt:
Step 1: Open Command Prompt.
Step 2: Go to Folder/Workspace where your project/files stored.
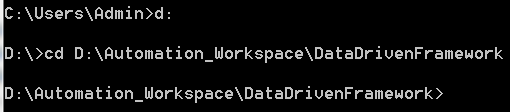
In my case my project is stored in D: Drive and User should be in Project Folder.
Step 3: git init >> Command to initialise project with repository.
Step 4: git status >> To check status.
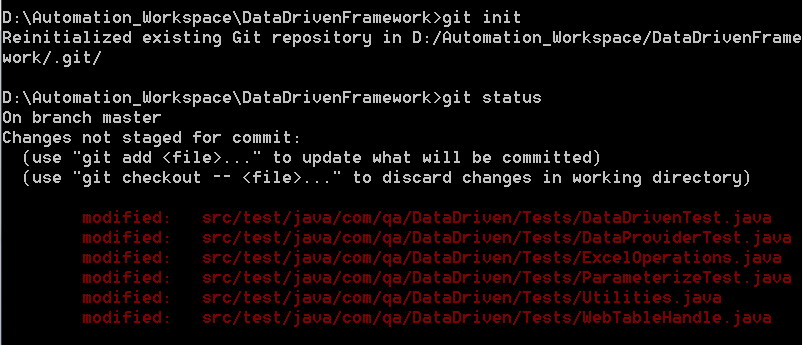
Since my project is already associated with created repository, it is showing as Reinitialized existing Git Repository. If it is not initialized, still you can proceed and you can initialize later on.
Then I am checking status of files >> All files are highlighted in Red color because they are not ready to be committed.
Step 5: git add . Or git add Filename >> Commands to add all files or To add particular File.
Step 6: git status >> To check status.
Now all files are highlighted in Green color because all files are ready to be Committed.
Step 7: git commit –m “Comment Message”
Step 8: git status

Now my code is ready to be pushed to Repository.
If Project is not initialised with GIT Repository – Follow below command to initialise project with GIT Repository before pushing code to Repository. [It is a One Time Activity].
If Project is already initialzed you can ignore Step-9.
Step 9: git remote add origin “Path of Git Repository”
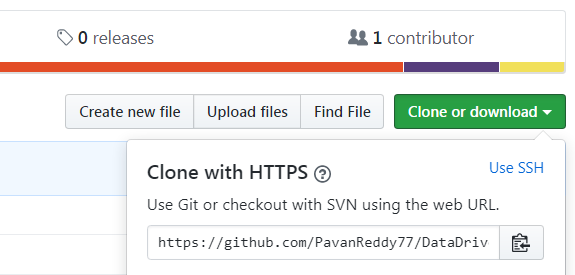
Now Project will be initialised with GIT Repository and we are good to push our code.
Step 10: git push origin master [Master >> Branch Name].
If it asks for credentials >> Enter Valid Username and Password.
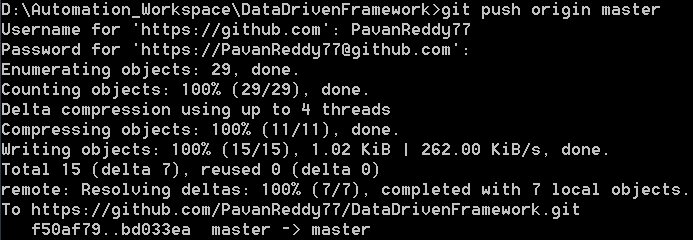
Code is Pushed to initialised GIT Repository.
Other git commands:
git clone “repository path“ – To download or clone complete repository from git.
git pull origin master – Once repository is cloned from git to local and we udpate any code for same repository in git. To get same code to be udpated in Local and in Eclipse we can use this command.
This blog is written by Pavan. He is an expert in Selenium Automation and currently working in Infosys as Automation QA Engineer.
Cheers!
Naveen AutomationLabs