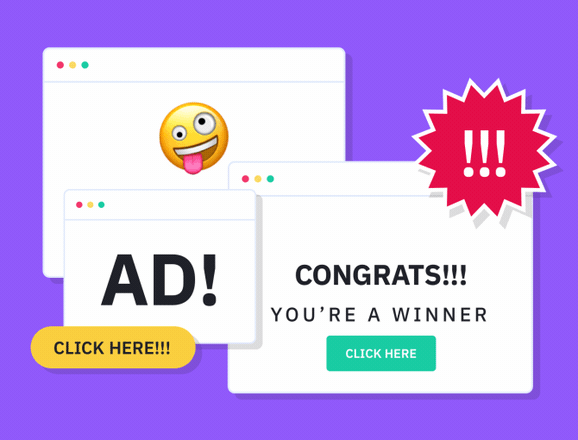
1. Overview
In this article, we will discuss the concept of browser window popup also the practical implementation to handle the browser window popup with selenium web driver.
2. Browser window Popup
- Browser window popup is a window popup that suddenly pops up on after clicking on some link or on selecting an option. It just likes another web page where maximization and minimization of the web page can be done.
- This is having its own title and url or it could be an advertisement pop up also.
- When do we need to handle this browser window popup: if the scenario is something like: you click on some link on the main window (parent window) and some other screen pops out just another webpage (child window ), now child window demands to submit some important information and press OK once information is filled.
- Let’s see how the browser window appears:
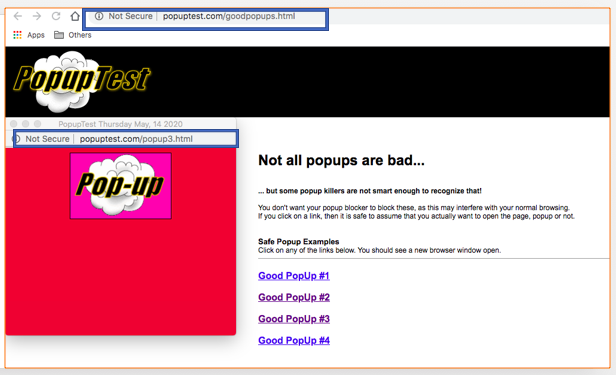
Browser window popup Example
3. Steps to deal with browser window popup
1. Operate on the main window that opens the popup window. For instance, click on some link on the main window.
2. Need to know the name or id of the popup window. The window Id can be fetched from Window Handler API available in selenium. it uniquely identifies the address of all the windows open at a time. Below are the methods to handle the windows:
Syntax:
driver.getWindowHandle();
- This function allows the handling of the current window by getting the current window Handle.
- It returns the type String.
- On the selenium official site, this is defined as

String parentWindowObj = driver.getwindowhandle();
driver.getwindowhandles();
- As the method name suggests windowHandles, that means there are multiple windows and to handle all these opened windows we have to use this function.
- It returns the Set of String.
-
Set<String> handles = driver.getwindowhandles();
-
- Set stores the unique values and handle of all the windows that will be unique.
- Below description clearly says the same:

- Set values are not stored based on the index, so to iterate over the set, we need to use the Iterator to get the handler values and this method will give you an iterator of string.
- This Iterator will be available in java. util package.
Iterator<String> it = handler.iterator();
//get the window ID for Parent a& Child now
String parentWindowID = it.next();
String childWindowID = it.next();
3. switch the driver to the popup window
driver.switchTo.window(childWindowID);
4. Perform some actions in the popup window such as get the title or close the button/tab etc.
driver.switchTo().window(childWindowID);
String title = driver.getTitle();
System.out.println("child window title is : " + title);
//close the pop up window now by using driver.close() method.
driver.close();
tip: Make sure, you are using close() to close the pop up. If you use quit() it will close parent and child both.
This is the basic difference between close() & quit().
5. Switch back to the parent window by passing the parentWindowID.
driver.switchTo().window(parentWindowID);
String title = driver.getTitle();
System.out.println("parent window title is : " + title);
//close the parent window by using quit:
driver.quit();
Let’s see the below code for a complete understanding of the concept:
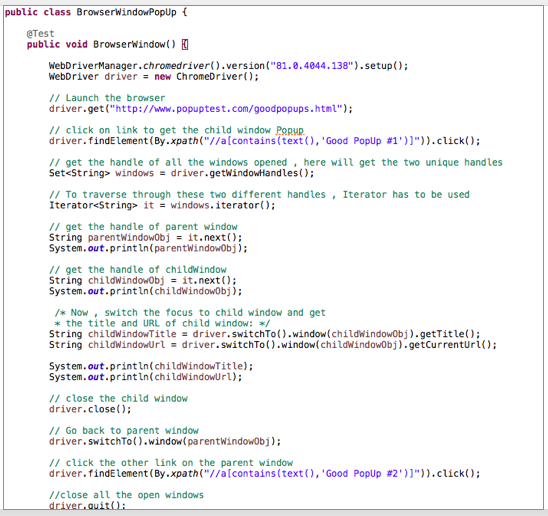
package SeleniumSessions;
import java.util.Iterator;
import java.util.Set;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import io.github.bonigarcia.wdm.WebDriverManager;
public class BrowserWindowPopUp {
public static void main(String[] args) {
WebDriverManager.chromedriver().setup();
WebDriver driver = new ChromeDriver();
driver.get(“http://www.popuptest.com/goodpopups.html”);
driver.findElement(By.linkText(“Good PopUp #3”)).click();
Set<String> handles = driver.getWindowHandles();
Iterator<String> it = handles.iterator();
String parentWindowID = it.next();
System.out.println(“parent window id is : ” + parentWindowID);
String childWindowID = it.next();
System.out.println(“child pop up window id is : ” + childWindowID);
driver.switchTo().window(childWindowID);
System.out.println(“child window title is ” + driver.getTitle());
driver.close();
driver.switchTo().window(parentWindowID);
System.out.println(“parent window title is ” + driver.getTitle());
}
}
Important Tip:
One thing to notice here is that we have to come back to parent window if any further actions have to performed on Parent window. Without switching to parent window will not allow us to perform any action on parent window page.
Let’s add another example to get more understanding of the concept:
Suppose four windows are open and we want to switch to the third window.
- First Approach: using Set and iterator we have already seen above.
- Second Approach: Using ArrayList. The benefit of this approach is you can easily deal with any window. The parent window will start with an index 0.
Let’s see the code with ArrayList:
package SeleniumSessions;
import java.util.ArrayList;
import java.util.Set;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class BrowserWindowHandleList {
public static void main(String[] args) {
System.setProperty(“webdriver.chrome.driver”, “/Users/NaveenKhunteta/Downloads/chromedriver”);
WebDriver driver = new ChromeDriver();
driver.get(“http://www.popuptest.com/goodpopups.html”);
driver.findElement(By.linkText(“Good PopUp #3”)).click();
Set<String> handles = driver.getWindowHandles();
ArrayList<String> ar = new ArrayList<String>(handles);
System.out.println(ar);
String parentWindowID = ar.get(0);
System.out.println(parentWindowID);
String childWindowID = ar.get(1);
System.out.println(childWindowID);
driver.switchTo().window(childWindowID);
System.out.println(“child window title is “ + driver.getTitle());
driver.close();
driver.switchTo().window(parentWindowID);
System.out.println(“parent window title is “ + driver.getTitle());
}
}
And we’ll get the output as following:
As can be seen through the above code, you can easily browse through the windows following this approach.
6. Conclusion
We have learnt to handle browser window popup using windowhandler API in Selenium WebDriver. We have solved this use cases by using Set and List both.
That’s about it.
Cheers!!
Naveen AutomationLabs
Blog Contributors:
Author: Mandeep Kaur
Mandeep, having 5+ years of Testing experience in automation using Selenium (Java). Expertise in API and Performance testing using JMeter.
https://www.linkedin.com/in/mandeepkaur93
Reviewer: Naveen Khunteta
https://www.linkedin.com/in/naveenkhunteta
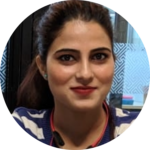
Leave a Reply